Unity笔记-物品管理器
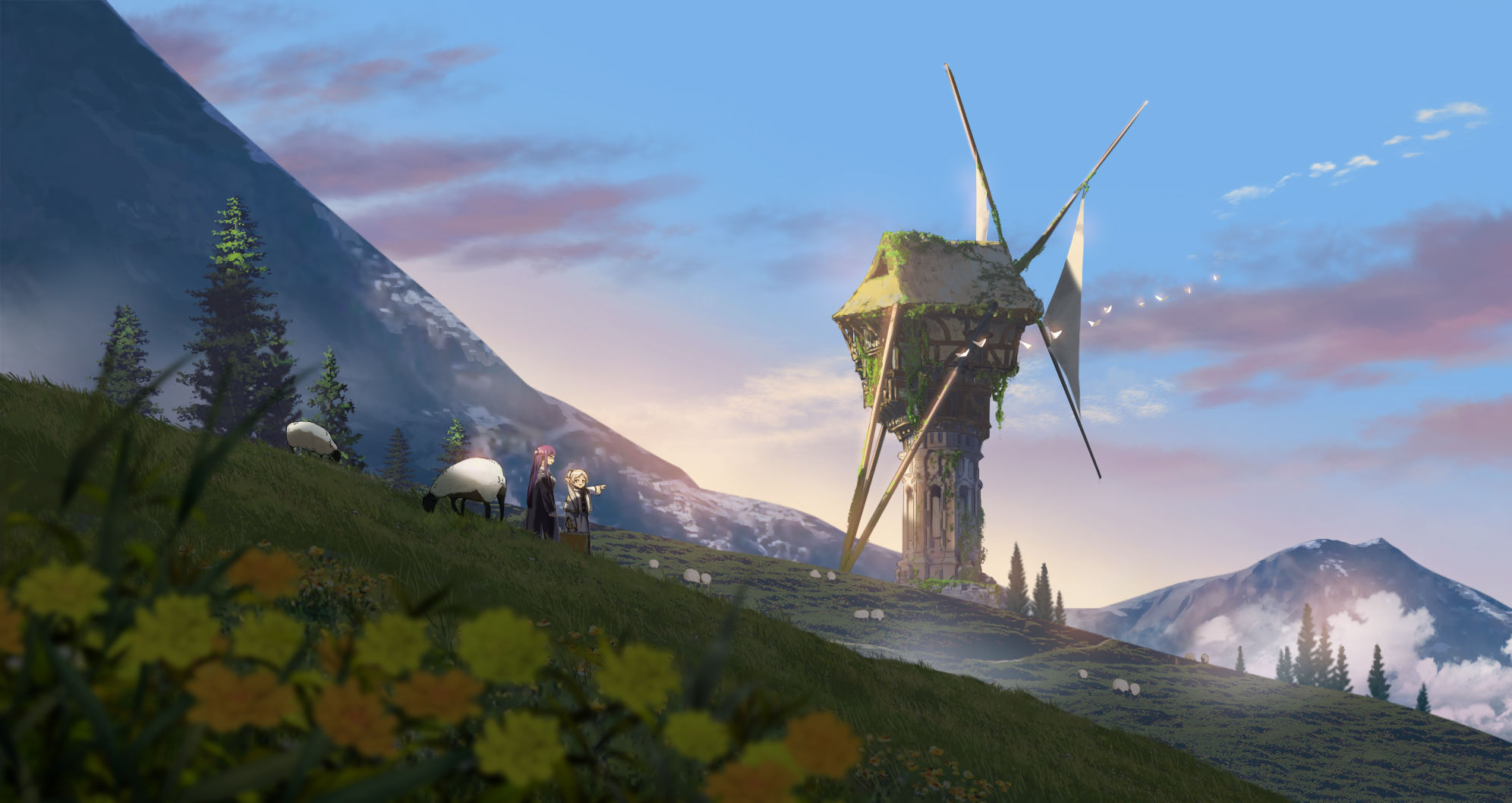
Unity笔记-物品管理器
Wang ChenHan准备:LitJson插件(Unity笔记-json和xml)
使用Json来存储物品信息
- 创建Resources文件夹(注意文件夹名字一定要是Resources)
右键Resources文件夹,选择Show in Explorer以资源管理器打开文件夹。
在Resources文件夹里创建Item.json文件
打开Item.json文件,输入以下json:然后打开ItemManager脚本,编写以下代码:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16[
{
"id":1,
"name":"宝剑",
"des":"这是一把平平无奇的剑",
"price":200,
"attack":10
},
{
"id":2,
"name":"弓箭",
"des":"一把某人用过的弓箭",
"price":900,
"attack":50
}
]1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40//using...
using LitJson;
public class Item
{
public int id;
public string name;//要与json对应
public string des;
public int price;
public string icon;
public int attack;
}
public class ItemManager
{
private static ItemManager instance;
public static ItemManager Instance
{
get{
if(instance==null){
instance = new ItemManager();
}
return instance;}
}
//保存所有物品
private Item[] item;
public ItemManager(){
//加载json数据
TextAsset json = Resources.Load<TextAsset>("item");
//解析json
item = LitJson.JsonMapper.ToObject<Item[]>(json.text);
}
//取物品
public Item GetItem(int id){
foreach(Item i in item){
if(i.id == id){
return i;
}
}
return null;
}
}
评论
匿名评论隐私政策
✅ 你无需删除空行,直接评论以获取最佳展示效果